http://examples.javacodegeeks.com/android/core/ui/webview/android-webview-example/
In Android, you can use the WebView component in order to present markup pages from URL or custom html markup page (short of like a browser, but in your own application…). In this tutorial we are going to create an use two Activities. The main Activity will contain a button. When the user presses that button, another activity launches which will contain a web view and will present the Home Page of JavaCodeGeeks.
For this tutorial, we will use the following tools in a Windows 64-bit platform:
- JDK 1.7
- Eclipse 4.2 Juno
- Android SKD 4.2
1. Create a new Android Project
Open Eclipse IDE and go to File -> New -> Project -> Android -> Android Application Project and click Next.

You have to specify the Application Name, the Project Name and the Package name in the appropriate text fields and then click Next.

In the next window make sure the “Create activity” option is selected in order to create a new activity for your project, and click Next. This is optional as you can create a new activity after creating the project, but you can do it all in one step.

Select “BlankActivity” and click Next.

You will be asked to specify some information about the new activity. In the Layout Name text field you have to specify the name of the file that will contain the layout description of your app. In our case the file res/layout/main.xml
will be created. Then, click Finish.

2. Create the Layout of the main Activity
Open res/layout/main.xml
file :

And paste the following code :
01 | <? xml version = "1.0" encoding = "utf-8" ?> |
03 | android:layout_width = "fill_parent" |
04 | android:layout_height = "fill_parent" |
05 | android:orientation = "vertical" > |
08 | android:id = "@+id/buttonUrl" |
09 | android:layout_width = "wrap_content" |
10 | android:layout_height = "wrap_content" |
11 | android:text = "JavaCodeGeeks Home Page" /> |
Now you may open the Graphical layout editor to preview the User Interface you created:

3. Create a new Activity
Go to the Package Explorer and right click on the package (in our case com.javacodegeeks.android.androidwebviewexample). Select New -> Class. Put the appropriate attributes as shown in the picture below:

As you will see a new java file has been created:
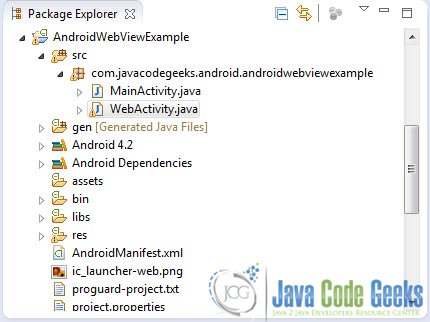
4. Create the Layout of the new activity
To create a new layout file, go to the Package Explorer and right click on the res/layout
folder. Select New -> Other -> Android -> Android XML Layout File. And click Next:

Then specify the name of the file and the Layout type and click Finish:
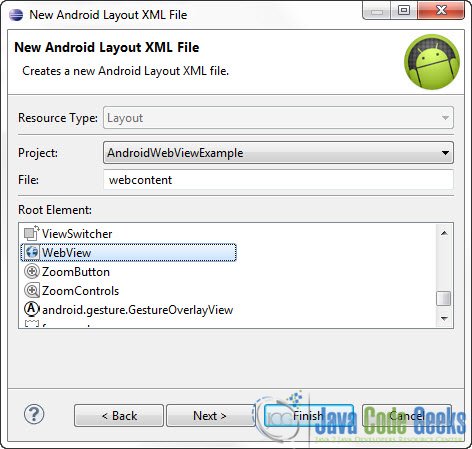
As you will see in the Package Explorer the new /res/layout/webcontent.xml
file has been created:

Open that file and paste the following code:
1 | <? xml version = "1.0" encoding = "utf-8" ?> |
3 | android:id = "@+id/webView" |
4 | android:layout_width = "fill_parent" |
5 | android:layout_height = "fill_parent" /> |
5. Code
Open WebActivity.java
and paste the following code:
01 | package com.javacodegeeks.android.androidwebviewexample; |
03 | import android.app.Activity; |
04 | import android.os.Bundle; |
05 | import android.webkit.WebView; |
07 | public class WebActivity extends Activity { |
09 | private WebView webView; |
11 | public void onCreate(Bundle savedInstanceState) { |
13 | super .onCreate(savedInstanceState); |
14 | setContentView(R.layout.webcontent); |
16 | webView = (WebView) findViewById(R.id.webView); |
18 | webView.getSettings().setJavaScriptEnabled( true ); |
Open MainActivity.java
and paste the following code:
01 | package com.javacodegeeks.android.androidwebviewexample; |
03 | import android.app.Activity; |
04 | import android.content.Context; |
05 | import android.content.Intent; |
06 | import android.os.Bundle; |
07 | import android.view.View; |
08 | import android.view.View.OnClickListener; |
09 | import android.widget.Button; |
11 | public class MainActivity extends Activity { |
13 | private Button button; |
15 | public void onCreate(Bundle savedInstanceState) { |
16 | final Context context = this ; |
18 | super .onCreate(savedInstanceState); |
19 | setContentView(R.layout.main); |
21 | button = (Button) findViewById(R.id.buttonUrl); |
23 | button.setOnClickListener( new OnClickListener() { |
26 | public void onClick(View arg0) { |
27 | Intent intent = new Intent(context, WebActivity. class ); |
28 | startActivity(intent); |
6. Edit AndroidManifest.xml
When you want to display Internet content in your Application you need to set the appropriate permissions in yourAndroidManifest.xml
. You can do this by adding the followint line in AndroidManifest.xml
:
1 | < uses-permission android:name = "android.permission.INTERNET" /> |
We also need to declare our new Activity. So, open AndroidManifest.xml
:

And paste the following code:
01 | <? xml version = "1.0" encoding = "utf-8" ?> |
03 | package = "com.javacodegeeks.android.androidwebviewexample" |
04 | android:versionCode = "1" |
05 | android:versionName = "1.0" > |
07 | < uses-sdk android:minSdkVersion = "8" android:targetSdkVersion = "16" /> |
09 | < uses-permission android:name = "android.permission.INTERNET" /> |
12 | android:allowBackup = "true" |
13 | android:icon = "@drawable/ic_launcher" |
14 | android:label = "@string/app_name" |
15 | android:theme = "@style/AppTheme" > |
17 | android:name = ".WebActivity" |
18 | android:theme = "@android:style/Theme.NoTitleBar" /> |
21 | android:name = "com.javacodegeeks.android.androidwebviewexample.MainActivity" |
22 | android:label = "@string/app_name" > |
24 | < action android:name = "android.intent.action.MAIN" /> |
26 | < category android:name = "android.intent.category.LAUNCHER" /> |
7. Run the application
This is the main screen of our Application:

Now, when you press the button:

8. Load custom markup
Open WebActivity.java
and un-comment the last two lines:
01 | package com.javacodegeeks.android.androidwebviewexample; |
03 | import android.app.Activity; |
04 | import android.os.Bundle; |
05 | import android.webkit.WebView; |
07 | public class WebActivity extends Activity { |
09 | private WebView webView; |
11 | public void onCreate(Bundle savedInstanceState) { |
13 | super .onCreate(savedInstanceState); |
14 | setContentView(R.layout.webcontent); |
16 | webView = (WebView) findViewById(R.id.webView); |
18 | webView.getSettings().setJavaScriptEnabled( true ); |
22 | String customHtml = "<html><body><h2>Greetings from JavaCodeGeeks</h2></body></html>" ; |
23 | webView.loadData(customHtml, "text/html" , "UTF-8" ); |
9. Run the application
Now, when you press the button on the main sceen:

Download Eclipse Project
This was an Android WebView Example. Download the Eclipse Project of this tutorial: AndroidWebViewExample.zip